Creating a React 18 Boilerplate Part 1 - Node, React and Tailwind installation Guide
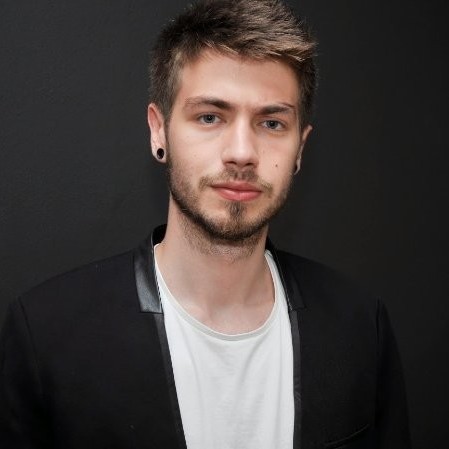
By Luka Lazic
10/08/2024
Initial Installation steps
First, we are going to install the latest supported node version, which in the moment of building this was v22.6.0. This should work with future versions as well, with very little if any difference. To install it, just download it from the nodejs website. The next step is to open up a console and type in npm create vite@latest react-19
, which will create a new folder react-19
. During the installation process, we pick React
as the framework and Typescript
as the variant. When all is installed, we can run npm run dev
and we should see the starting screen.
![[Pasted image 20240810134504.png]]
I like to run my app on port 3000 as most of my automation is based around that port, so I change vite.config.ts
to this which will run the app on the desired port.
import { defineConfig } from "vite";
import react from "@vitejs/plugin-react";
// https://vitejs.dev/config/
export default defineConfig({
plugins: [react()],
server: {
port: 3000,
},
});
Installing Tailwind
Next we want to add Tailwind, so we just install it standardly by using the command npm install -D tailwindcss postcss autoprefixer
which will install tailwind and the required packages. After it installs, we need to add the config files by typing npx tailwindcss init -p
, which will create postcss.config.js
as well ass tailwind.config.js
. At the time of writing, I am using Tailwind v3.4.9.
The tailwind config needs to be changed by adding the required content, which will basically be javascript and jsx files as well as their typed versions:
/** @type {import('tailwindcss').Config} */
export default {
content: [
"./index.html",
"./src/**/*.{js,ts,jsx,tsx}",
],
theme: {
extend: {},
},
plugins: [],
}
In App.css, we replace everything with the following:
@tailwind base;
@tailwind components;
@tailwind utilities;
By default, the template already imports the css in App.tsx, so we can now test it out and just replace the whole file with:
import "./App.css";
function App() {
return (
<div>
<h1 className="text-3xl font-bold text-center">Test Tailwind</h1>
</div>
);
}
export default App;
If the text is large, bolded and centered, we are good to go! It's also good practice to delete the unused src/index.css
css file since we are no longer using it. In the next article, I am going to cover the react-query setup with examples, and the link will be posted here.